Metadata World Gen! Beta 1.7.3
This is more of an addition than a mod.
Though this mod adds a class I've named 'WorldGenMinableMetadata' which is basically the same as the 'WorldGenMinable' class expect it allows for block metadata to pass through and in turn generate in the world.
Two Versions
Spoiler
I have added two different versions of both client and server in the downloads section. The versions that say "+ Example Mod" include a small Modloader, ModloaderMP Mod that adds in generation code for each metadata color of wool. This mod is by no means a requiremeant it was simply added for people who maybe don't understand or need a example I will include it down below and you can compile it yourself if you'd like.
If you choose the version without the example mod you will not need modloader.
This example mod does require Modloader Beta 1.7.3 and ModloaderMP Unofficial v2. This video will show you have to navigate MCArchive and how to download those APIs. MCArchive: Best Place To Get Beta 1.7.3 Mods/APIs
WorldGenMinableMetadata class
Spoiler
package net.minecraft.src;
import java.util.Random;
public class WorldGenMinableMetadata extends WorldGenerator {
private int minableBlockId;
private int numberOfBlocks;
private int blockMetadata;
public WorldGenMinableMetadata(int i1, int i2, int i3) {
this.minableBlockId = i1;
this.blockMetadata = i2;
this.numberOfBlocks = i3;
}
public boolean generate(World world1, Random random2, int i3, int i4, int i5) {
float f6 = random2.nextFloat() * (float)Math.PI;
double d7 = (double)((float)(i3 + 8) + MathHelper.sin(f6) * (float)this.numberOfBlocks / 8.0F);
double d9 = (double)((float)(i3 + 8) - MathHelper.sin(f6) * (float)this.numberOfBlocks / 8.0F);
double d11 = (double)((float)(i5 + 8) + MathHelper.cos(f6) * (float)this.numberOfBlocks / 8.0F);
double d13 = (double)((float)(i5 + 8) - MathHelper.cos(f6) * (float)this.numberOfBlocks / 8.0F);
double d15 = (double)(i4 + random2.nextInt(3) + 2);
double d17 = (double)(i4 + random2.nextInt(3) + 2);
for(int i19 = 0; i19 <= this.numberOfBlocks; ++i19) {
double d20 = d7 + (d9 - d7) * (double)i19 / (double)this.numberOfBlocks;
double d22 = d15 + (d17 - d15) * (double)i19 / (double)this.numberOfBlocks;
double d24 = d11 + (d13 - d11) * (double)i19 / (double)this.numberOfBlocks;
double d26 = random2.nextDouble() * (double)this.numberOfBlocks / 16.0D;
double d28 = (double)(MathHelper.sin((float)i19 * (float)Math.PI / (float)this.numberOfBlocks) + 1.0F) * d26 + 1.0D;
double d30 = (double)(MathHelper.sin((float)i19 * (float)Math.PI / (float)this.numberOfBlocks) + 1.0F) * d26 + 1.0D;
int i32 = MathHelper.floor_double(d20 - d28 / 2.0D);
int i33 = MathHelper.floor_double(d22 - d30 / 2.0D);
int i34 = MathHelper.floor_double(d24 - d28 / 2.0D);
int i35 = MathHelper.floor_double(d20 + d28 / 2.0D);
int i36 = MathHelper.floor_double(d22 + d30 / 2.0D);
int i37 = MathHelper.floor_double(d24 + d28 / 2.0D);
for(int i38 = i32; i38 <= i35; ++i38) {
double d39 = ((double)i38 + 0.5D - d20) / (d28 / 2.0D);
if(d39 * d39 < 1.0D) {
for(int i41 = i33; i41 <= i36; ++i41) {
double d42 = ((double)i41 + 0.5D - d22) / (d30 / 2.0D);
if(d39 * d39 + d42 * d42 < 1.0D) {
for(int i44 = i34; i44 <= i37; ++i44) {
double d45 = ((double)i44 + 0.5D - d24) / (d28 / 2.0D);
if(d39 * d39 + d42 * d42 + d45 * d45 < 1.0D && world1.getBlockId(i38, i41, i44) == Block.stone.blockID) {
world1.setBlockAndMetadata(i38, i41, i44, this.minableBlockId, this.blockMetadata);
}
}
}
}
}
}
}
return true;
}
}
mod_WorldGenMetadataExample class
Spoiler
package net.minecraft.src;
import java.util.Random;
public class mod_WorldGenMetadataExample extends BaseModMp {
public mod_WorldGenMetadataExample() {
}
public void GenerateSurface(World world, Random random, int chunkX, int chunkZ) {
int posX;
int posY;
int posZ;
int i;
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 9, 16)).generate(world, random, posX, posY, posZ);
/*
* new WorldGenMinableMetadata is a copy of WorldGenMinable that has been edited to recognize metadata.
* Where it would normally be (new WorldGenMinable(Block.cloth.blockID, 16)).generate(world, random, posX, posY, posZ); and only give you White wool/cloth
* Now you can add the metadata to make it look like this (new WorldGenMinableMetadata(Block.cloth.blockID, 9, 16)).generate(world, random, posX, posY, posZ);
* The 9 is the metadata for that block, I believe this will generate Cyan wool in the world instead of White
*/
}
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 0, 16)).generate(world, random, posX, posY, posZ);
}
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 1, 16)).generate(world, random, posX, posY, posZ);
}
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 2, 16)).generate(world, random, posX, posY, posZ);
}
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 3, 16)).generate(world, random, posX, posY, posZ);
}
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 4, 16)).generate(world, random, posX, posY, posZ);
}
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 5, 16)).generate(world, random, posX, posY, posZ);
}
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 6, 16)).generate(world, random, posX, posY, posZ);
}
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 7, 16)).generate(world, random, posX, posY, posZ);
}
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 8, 16)).generate(world, random, posX, posY, posZ);
}
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 10, 16)).generate(world, random, posX, posY, posZ);
}
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 11, 16)).generate(world, random, posX, posY, posZ);
}
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 12, 16)).generate(world, random, posX, posY, posZ);
}
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 13, 16)).generate(world, random, posX, posY, posZ);
}
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 14, 16)).generate(world, random, posX, posY, posZ);
}
for(i = 0; i < 32; ++i) {
posX = chunkX + random.nextInt(16);
posY = random.nextInt(128);
posZ = chunkZ + random.nextInt(16);
(new WorldGenMinableMetadata(Block.cloth.blockID, 15, 16)).generate(world, random, posX, posY, posZ);
}
}
public String Version() {
return "v1.0";
}
public String Name() {
return "This is an example of how to use Metadata World Gen.";
}
}
Project members
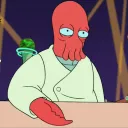
poopiedoopie9002
Member